来源: .NET开源最全的第三方登录整合库 – CollectiveOAuth – 追逐时光者 – 博客园
前言
我相信很多同学都对接过各种各样的第三方平台的登录授权获取用户信息(如:微信登录、支付宝登录、GitHub登录等等)。今天给大家推荐一个.NET开源最全的第三方登录整合库:CollectiveOAuth。
官方项目介绍
.Net平台(C#) 史上最全的整合第三方登录的开源库 => 环境支持 .NET Framework 4.5 ~ 4.6.2 和 .NetCore 3.1。目前已包含Github、Gitee、钉钉、百度、支付宝、微信、企业微信、腾讯云开发者平台(Coding)、OSChina、微博、QQ、Google、Facebook、抖音、领英、小米、微软、今日头条、Teambition、StackOverflow、Pinterest、人人、华为、酷家乐、Gitlab、美团、饿了么、等第三方平台的授权登录。
项目特点
- 全:已集成二十多家第三方平台(国内外常用的基本都已包含),仍然还在持续扩展中!
- 简:API就是奔着最简单去设计的(见后面快速开始),尽量让您用起来没有障碍感!
企业微信扫码授权快速开始
0、企业微信开发对接文档
文档介绍
企业微信扫码授权登录官方文档地址:https://developer.work.weixin.qq.com/document/path/91025,在进行企业微信扫码授权绑定/登录之前需要先自建应用,同时需要开启网页授权登录,具体自建应用的相关操作可以参考博文:https://developer.aliyun.com/article/1136114
管理平台接入
完成了上面企业微信管理后台的相关配置之后,我们就可以按照文档步骤开始操作了
构造二维码
关于构造企业微信扫码绑定/登录二维码一共有两种方式:构造独立窗口登录二维码、构造内嵌登录二维码,下面简单说一下构造独立窗口登录二维码
构造独立窗口登录二维码
构造独立窗口登录二维码,可以在页面放置一个 button 按钮,添加点击事件,在触发点击事件时访问连接https://open.work.weixin.qq.com/wwopen/sso/qrConnect?appid=CORPID&agentid=AGENTID&redirect_uri=REDIRECT_URI&state=STATE,效果如图:
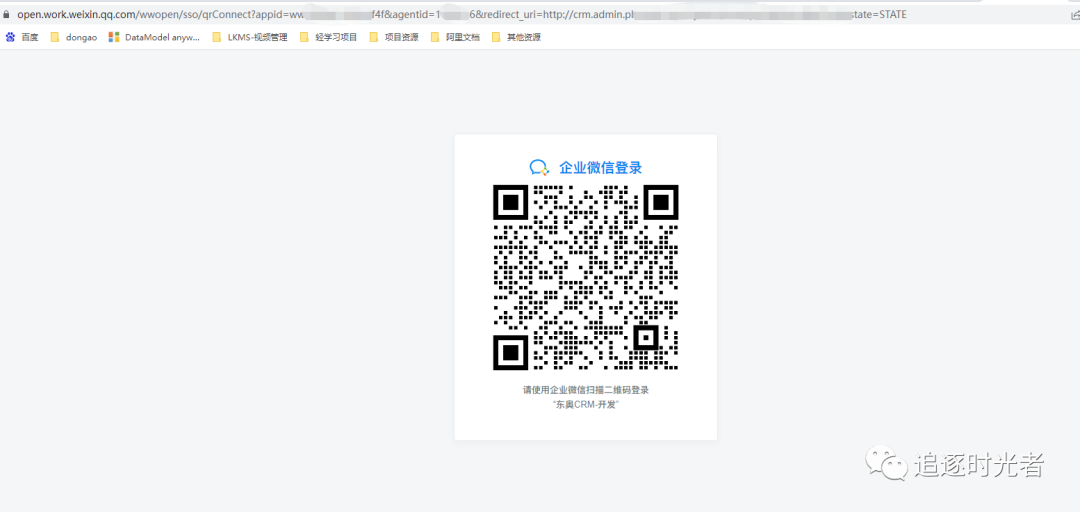
连接参数说明:
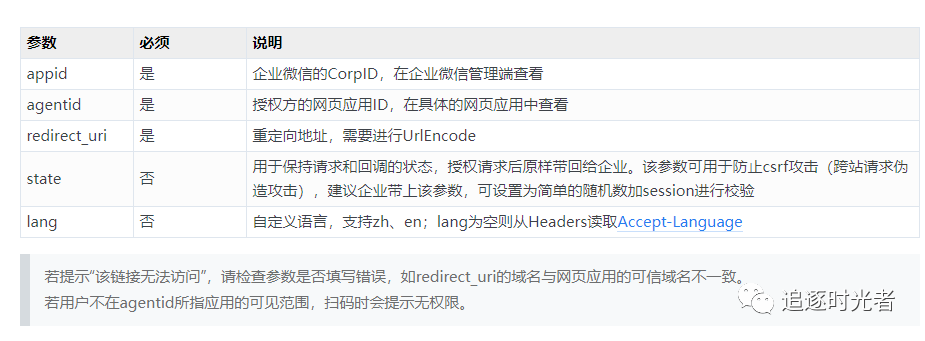
1、引入依赖
下载源码,添加Come.CollectiveOAuth类库。
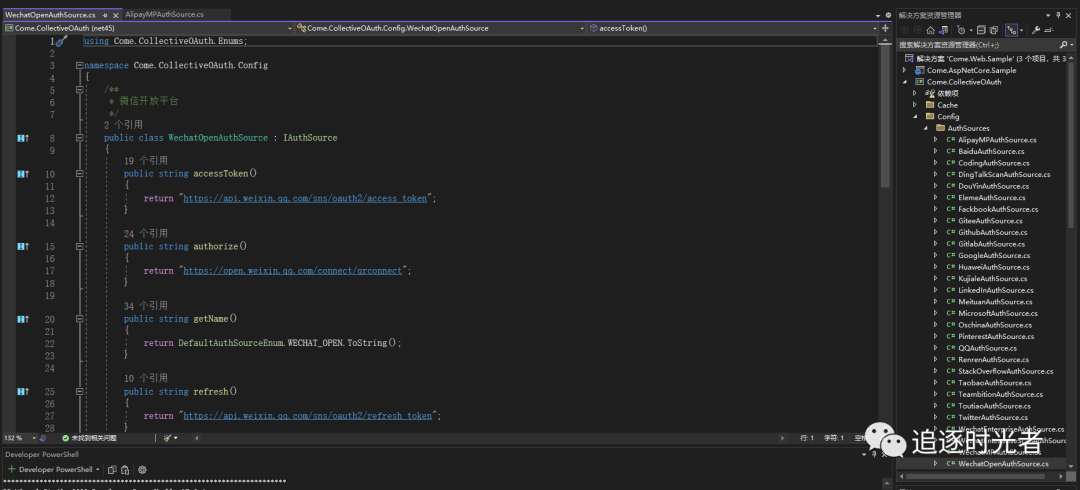
2、配置企业微信扫码授权(appsettings.json)中配置
{
"AppSettings": {
"CollectiveOAuth_WECHAT_ENTERPRISE_SCAN_ClientId": "xxxxxxxxxxxxxxxxx",
"CollectiveOAuth_WECHAT_ENTERPRISE_SCAN_AgentId": "xxxxxx",
"CollectiveOAuth_WECHAT_ENTERPRISE_SCAN_ClientSecret": "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx",
"CollectiveOAuth_WECHAT_ENTERPRISE_SCAN_RedirectUri": "https://yours.domain.com/oauth2/callback?authSource=WECHAT_ENTERPRISE_SCAN"
},
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft": "Warning",
"Microsoft.Hosting.Lifetime": "Information"
}
},
"AllowedHosts": "*"
}
构建授权Url方法
public IActionResult Authorization(string authSource= "WECHAT_ENTERPRISE_SCAN")
{
AuthRequestFactory authRequest = new AuthRequestFactory();
var request = authRequest.getRequest(authSource);
var authorize = request.authorize(AuthStateUtils.createState());
Console.WriteLine(authorize);
return Redirect(authorize);
}
public class AuthRequestFactory
{
#region 从Webconfig中获取默认配置(可以改造成从数据库中读取)
public Dictionary<string, ClientConfig> _clientConfigs;
public Dictionary<string, ClientConfig> ClientConfigs
{
get
{
if (_clientConfigs == null)
{
var _defaultPrefix = "CollectiveOAuth_";
_clientConfigs = new Dictionary<string, ClientConfig>();
#region 或者默认授权列表数据
var defaultAuthList = typeof(DefaultAuthSourceEnum).ToList().Select(a => a.Name.ToUpper()).ToList();
foreach (var authSource in defaultAuthList)
{
var clientConfig = new ClientConfig();
clientConfig.clientId = AppSettingUtils.GetStrValue($"{_defaultPrefix}{authSource}_ClientId");
clientConfig.clientSecret = AppSettingUtils.GetStrValue($"{_defaultPrefix}{authSource}_ClientSecret");
clientConfig.redirectUri = AppSettingUtils.GetStrValue($"{_defaultPrefix}{authSource}_RedirectUri");
clientConfig.alipayPublicKey = AppSettingUtils.GetStrValue($"{_defaultPrefix}{authSource}_AlipayPublicKey");
clientConfig.unionId = AppSettingUtils.GetStrValue($"{_defaultPrefix}{authSource}_UnionId");
clientConfig.stackOverflowKey = AppSettingUtils.GetStrValue($"{_defaultPrefix}{authSource}_StackOverflowKey");
clientConfig.agentId = AppSettingUtils.GetStrValue($"{_defaultPrefix}{authSource}_AgentId");
clientConfig.scope = AppSettingUtils.GetStrValue($"{_defaultPrefix}{authSource}_Scope");
_clientConfigs.Add(authSource, clientConfig);
}
#endregion
}
return _clientConfigs;
}
}
public ClientConfig GetClientConfig(string authSource)
{
if (authSource.IsNullOrWhiteSpace())
{
return null;
}
if (!ClientConfigs.ContainsKey(authSource))
{
return null;
}
else
{
return ClientConfigs[authSource];
}
}
#endregion
public IAuthRequest getRequest(string authSource)
{
IAuthRequest authRequest = getDefaultRequest(authSource);
return authRequest;
}
private IAuthRequest getDefaultRequest(string authSource)
{
ClientConfig clientConfig = GetClientConfig(authSource);
IAuthStateCache authStateCache = new DefaultAuthStateCache();
DefaultAuthSourceEnum authSourceEnum = GlobalAuthUtil.enumFromString<DefaultAuthSourceEnum>(authSource);
switch (authSourceEnum)
{
case DefaultAuthSourceEnum.WECHAT_MP:
return new WeChatMpAuthRequest(clientConfig, authStateCache);
case DefaultAuthSourceEnum.WECHAT_OPEN:
return new WeChatOpenAuthRequest(clientConfig, authStateCache);
case DefaultAuthSourceEnum.WECHAT_ENTERPRISE:
return new WeChatEnterpriseAuthRequest(clientConfig, authStateCache);
case DefaultAuthSourceEnum.WECHAT_ENTERPRISE_SCAN:
return new WeChatEnterpriseScanAuthRequest(clientConfig, authStateCache);
case DefaultAuthSourceEnum.ALIPAY_MP:
return new AlipayMpAuthRequest(clientConfig, authStateCache);
case DefaultAuthSourceEnum.GITEE:
return new GiteeAuthRequest(clientConfig, authStateCache);
case DefaultAuthSourceEnum.GITHUB:
return new GithubAuthRequest(clientConfig, authStateCache);
case DefaultAuthSourceEnum.BAIDU:
return new BaiduAuthRequest(clientConfig, authStateCache);
case DefaultAuthSourceEnum.XIAOMI:
return new XiaoMiAuthRequest(clientConfig, authStateCache);
case DefaultAuthSourceEnum.DINGTALK_SCAN:
return new DingTalkScanAuthRequest(clientConfig, authStateCache);
case DefaultAuthSourceEnum.OSCHINA:
return new OschinaAuthRequest(clientConfig, authStateCache);
case DefaultAuthSourceEnum.CODING:
return new CodingAuthRequest(clientConfig, authStateCache);
case DefaultAuthSourceEnum.LINKEDIN:
return new LinkedInAuthRequest(clientConfig, authStateCache);
case DefaultAuthSourceEnum.WEIBO:
return new WeiboAuthRequest(clientConfig, authStateCache);
case DefaultAuthSourceEnum.QQ:
return new QQAuthRequest(clientConfig, authStateCache);
case DefaultAuthSourceEnum.DOUYIN:
return new DouyinAuthRequest(clientConfig, authStateCache);
case DefaultAuthSourceEnum.GOOGLE:
return new GoogleAuthRequest(clientConfig, authStateCache);
case DefaultAuthSourceEnum.FACEBOOK:
return new FackbookAuthRequest(clientConfig, authStateCache);
case DefaultAuthSourceEnum.MICROSOFT:
return new MicrosoftAuthRequest(clientConfig, authStateCache);
case DefaultAuthSourceEnum.TOUTIAO:
return new ToutiaoAuthRequest(clientConfig, authStateCache);
case DefaultAuthSourceEnum.TEAMBITION:
return new TeambitionAuthRequest(clientConfig, authStateCache);
case DefaultAuthSourceEnum.RENREN:
return new RenrenAuthRequest(clientConfig, authStateCache);
case DefaultAuthSourceEnum.PINTEREST:
return new PinterestAuthRequest(clientConfig, authStateCache);
case DefaultAuthSourceEnum.STACK_OVERFLOW:
return new StackOverflowAuthRequest(clientConfig, authStateCache);
case DefaultAuthSourceEnum.HUAWEI:
return new HuaweiAuthRequest(clientConfig, authStateCache);
case DefaultAuthSourceEnum.KUJIALE:
return new KujialeAuthRequest(clientConfig, authStateCache);
case DefaultAuthSourceEnum.GITLAB:
return new GitlabAuthRequest(clientConfig, authStateCache);
case DefaultAuthSourceEnum.MEITUAN:
return new MeituanAuthRequest(clientConfig, authStateCache);
case DefaultAuthSourceEnum.ELEME:
return new ElemeAuthRequest(clientConfig, authStateCache);
default:
return null;
}
}
}
API列表
https://gitee.com/rthinking/CollectiveOAuth#api%E5%88%97%E8%A1%A8
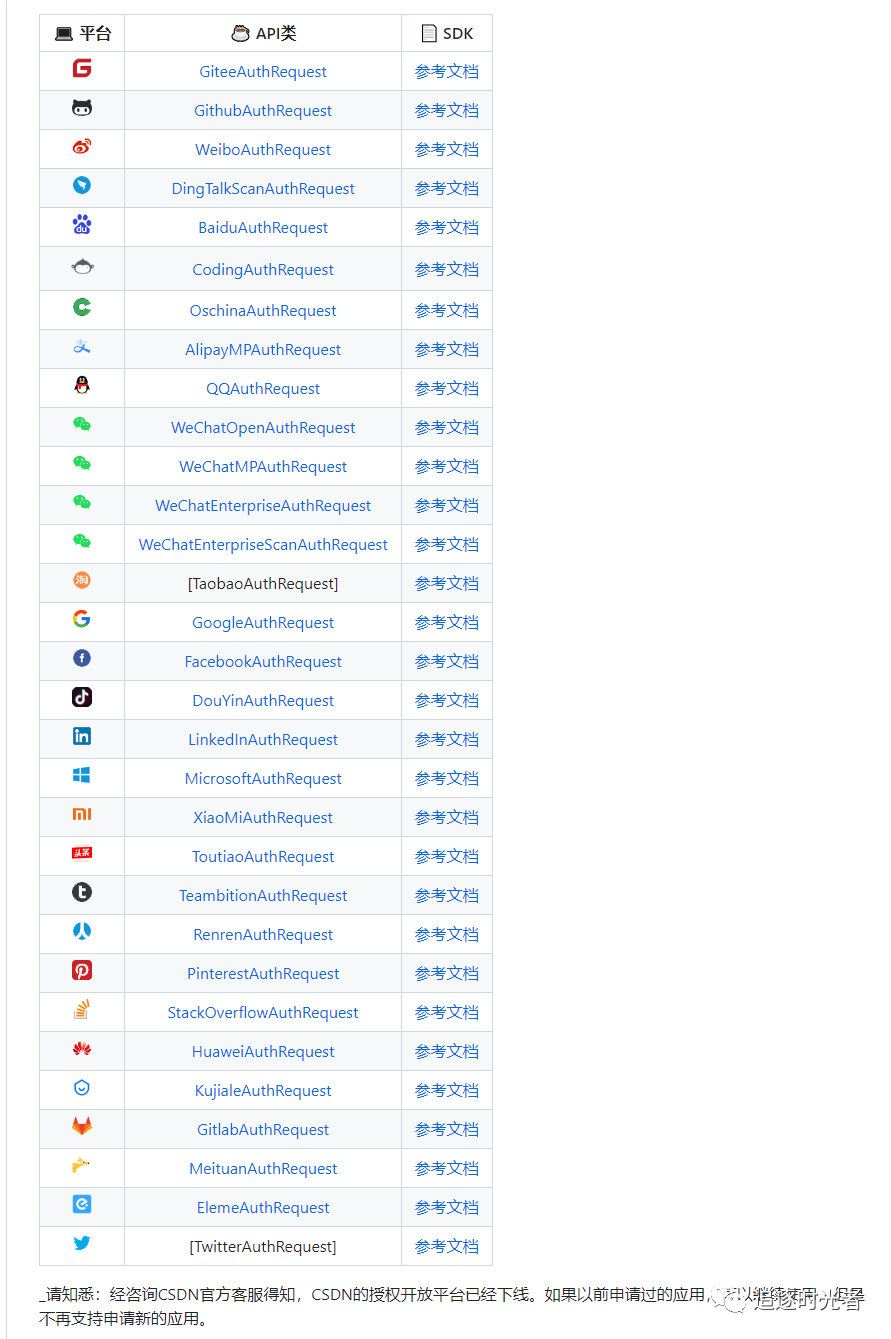
项目源码地址
更多实用功能特性欢迎前往开源地址查看👀,别忘了给项目一个Star💖。
https://gitee.com/rthinking/CollectiveOAuth
优秀项目和框架精选
该项目已收录到C#/.NET/.NET Core优秀项目和框架精选中,关注优秀项目和框架精选能让你及时了解C#、.NET和.NET Core领域的最新动态和最佳实践,提高开发效率和质量。坑已挖,欢迎大家踊跃提交PR,自荐(让优秀的项目和框架不被埋没🤞
)。
https://github.com/YSGStudyHards/DotNetGuide/blob/main/docs/DotNet/DotNetProjectPicks.md
加入DotNetGuide技术交流群
- 提供.NET开发者分享自己优质文章的群组和获取更多全面的C#/.NET/.NET Core学习资料、视频、文章、书籍,社区组织,工具和常见面试题资源,帮助大家更好地了解和使用 .NET技术。
- 在这个群里,开发者们可以分享自己的项目经验、遇到的问题以及解决方案,倾听他人的意见和建议,共同成长与进步。
- 可以结识更多志同道合的开发者,甚至可能与其他开发者合作完成有趣的项目。通过这个群组,我们希望能够搭建一个积极向上、和谐友善的.NET技术交流平台,为广大.NET开发者带来更多的价值。